Introduction
Happy π Day 2021! In this article I’ll estimate the digits of π with random numbers and the probability of two integers being co-prime. What is the probability of two random integers being coprime? Euclidean’s Algorithm can be used to estimate π!
Probability of Two Integers Being Coprime
Let
Now,
implying that
Or denoted in a statistical way
Euclidean Algorithm
In mathematics, the Euclidean Algorithm, or Euclid’s Algorithm, is an efficient method for computing the greatest common divisor (GCD) of two integers (numbers), the largest number that divides them both without a remainder.
Greatest Common Divisor
The greatest common divisor g is the largest natural number that divides both a and b without leaving a remainder.
def gcd(a, b):
while b != 0:
t = b
b = a % b
a = t
return a
Estimate Pi
Let’s step back to the probability of two integers being coprime. We now know that
it can be written as
so we get
Main Code
Talk is cheap. Show me the code.
iterations = 400
num_total = 0
num_coprime = 0
pies = []
for idx in range(iterations):
for i in range(500):
a, b = random.randint(1, 1000000), random.randint(1, 1000000)
if gcd(a, b) == 1:
num_coprime += 1
num_total += 1
pi = math.sqrt(6 * num_total / num_coprime)
pies.append(pi)
pi_average = sum(pies)/len(pies)
print(pi_average)
Visualise
Let’s visualise it dynamicly.
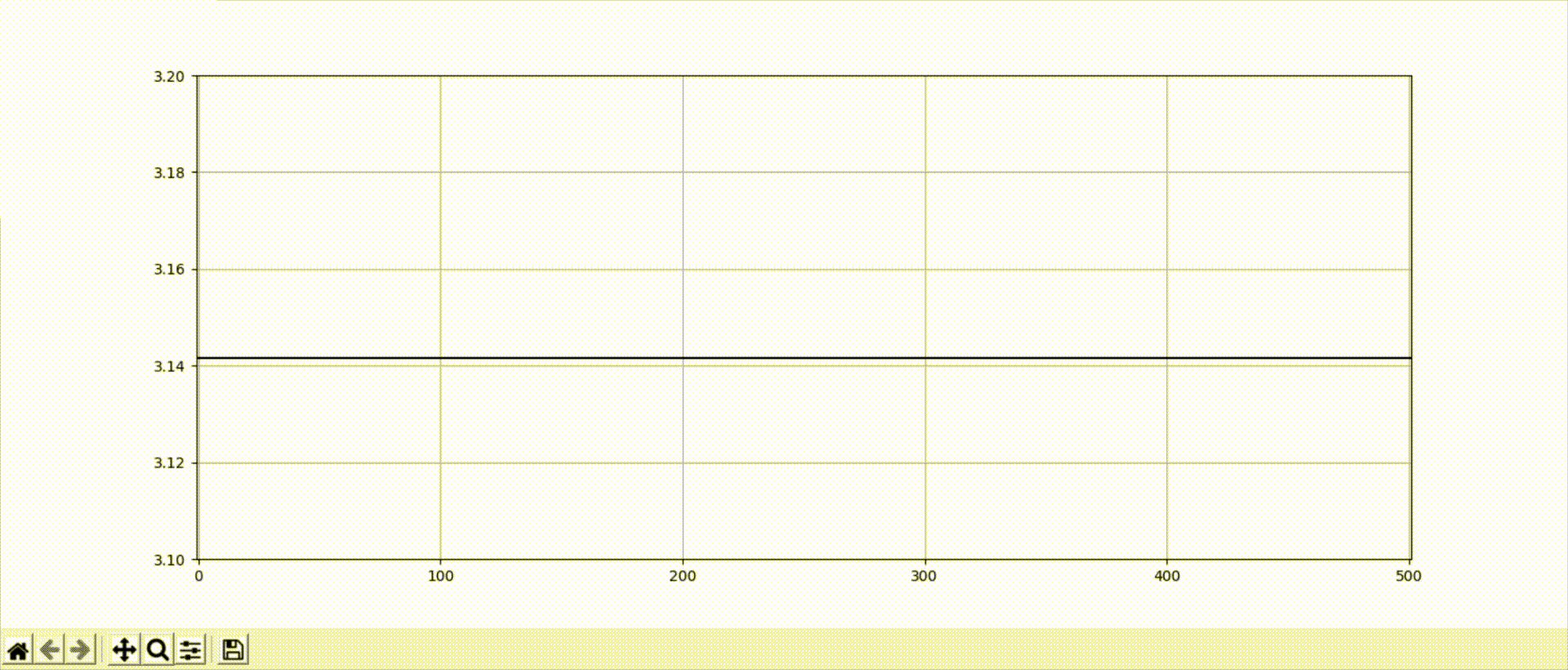
iterations = 400
num_total = 0
num_coprime = 0
pies = []
plt.rcParams["figure.figsize"] = (15, 6)
ax = plt.axes()
plt.xlim(-1, iterations+1)
plt.ylim(3, 3.3)
plt.axhline(y=3.1415, color='black', linestyle='-')
plt.grid()
for idx in range(iterations):
for i in range(500):
a, b = random.randint(1, 1000000), random.randint(1, 1000000)
if gcd(a, b) == 1:
num_coprime += 1
num_total += 1
pi = math.sqrt(6 * num_total / num_coprime)
pies.append(pi)
pi_average = sum(pies)/len(pies)
ax.plot(
[idx], [pi_average],
linestyle='-', lw=2, marker='o', color='tab:blue', markersize=2, alpha=0.5
)
plt.draw()
custom_lines = [Line2D([0], [0], color='tab:blue', lw=1)]
plt.legend(custom_lines, [f"pi={pi_average:.10f}"], loc="upper right")
plt.pause(0.01)
plt.show()
Conclusion
Today I show you how to estimate numbers π from random numbers using Euclidean algorithm. Happy coding! Cheers!
References
- https://www.cut-the-knot.org/m/Probability/TwoCoprime.shtml
- https://en.wikipedia.org/wiki/Euclidean_algorithm
- https://thecodingtrain.com/CodingChallenges/161-pi-from-random.html
- https://www.youtube.com/watch?v=EvS_a921dBo
- https://www.youtube.com/watch?v=RZBhSi_PwHU
- https://www.youtube.com/watch?v=d-o3eB9sfls&list=PLCZeVeoafktVGu9rvM9PHrAdrsUURtLTo&index=54&ab_channel=3Blue1Brown